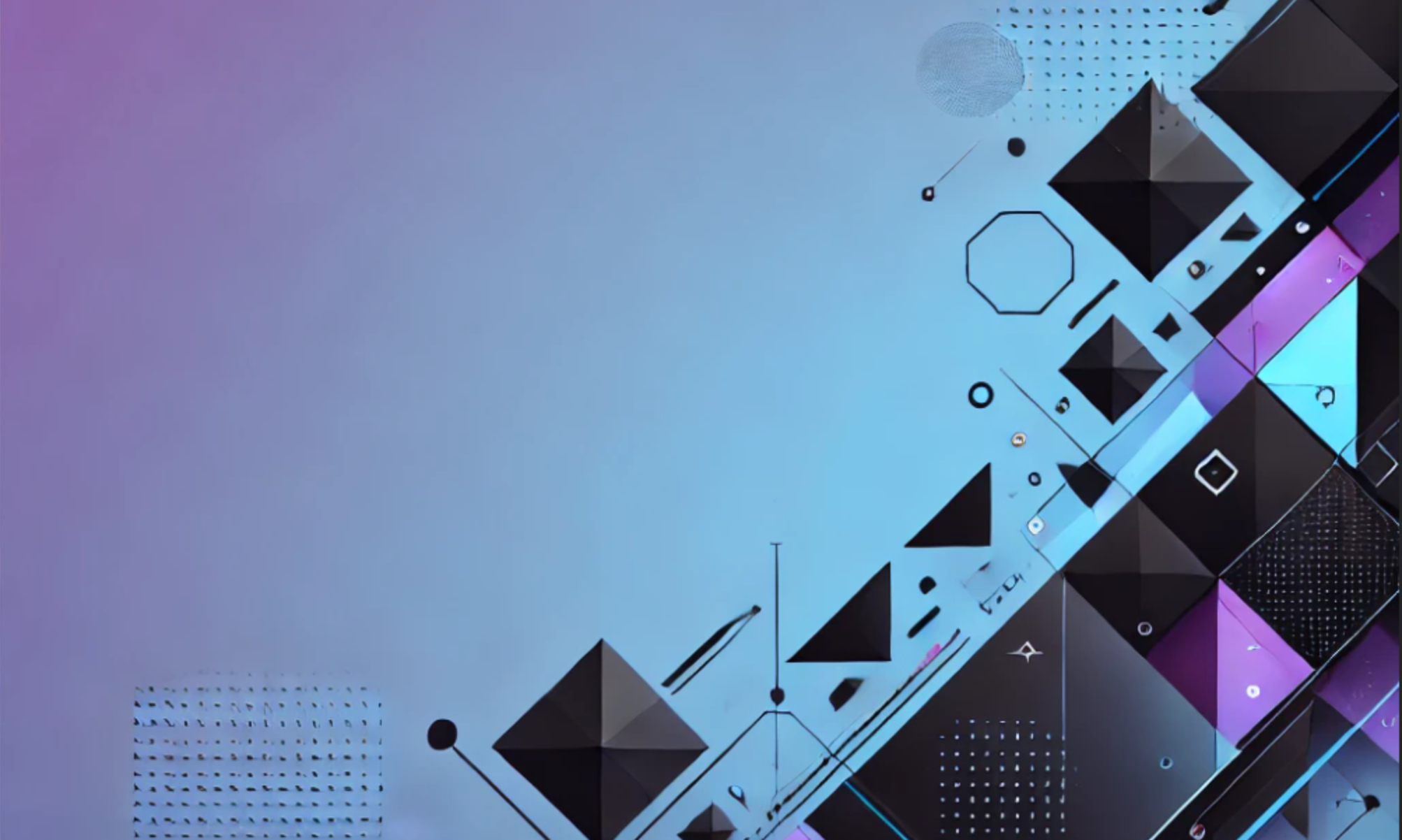
Simplify Localization in .NET with DotLocz
6 Min Read · 2024-11-05
Introduction
In large-scale projects, managing localization across multiple
modules and languages can quickly become complex and tedious. To
simplify this process, I developed DotLocz, a .NET tool
that makes localization easy, efficient, and even enjoyable by
converting .loc.csv
files into enums and
RESX files. This approach brings organization and
automation to localization, removing the manual handling that can bog
down development.
Why Choose DotLocz?
DotLocz was created out of a need for a flexible, AI-friendly localization tool to streamline localization for large, modular .NET projects. Here’s how DotLocz simplifies the localization workflow:
- Quick Setup: Start with a few
.loc.csv
files, and DotLocz takes care of the rest. - Automatic Enum and RESX Generation: Translations become strongly-typed resources—no manual work required.
- Supports Multiple Projects: Ideal for solutions
with multiple modules, DotLocz scans all
.loc.csv
files and organizes resources efficiently. - Effortless Language Expansion: CSV-based structure allows for easy autocompletion, making it smooth to add new languages.
Quick Example: Using DotLocz for Localization
Let’s walk through a basic example to see how DotLocz simplifies localization in .NET projects. Suppose we’re building a multilingual greeting feature.
Create a
.loc.csv
File: Define your translations in a file calledMessagesResources.loc.csv
.Key,en-US,fr-FR,es-ES Greeting,Hello,Bonjour,Hola Farewell,Goodbye,Au revoir,Adiós
Run DotLocz: Navigate to your project’s directory and run DotLocz to generate the RESX files and enums.
dot-locz . Locz
This command generates a folder named
Locz
, containing language-specific RESX files likeMessagesResources.en-US.resx
andMessagesResources.fr-FR.resx
, along with an enumMessagesResources
for referencing the keys.Access Resources in Code: Use the generated
MessagesResources
enum and a localizer in your code to retrieve localized strings. Here’s a simple example in a .NET component:using Microsoft.Extensions.Localization; using Locz; public class GreetingService { private readonly IStringLocalizer<MessagesResources> _localizer; public GreetingService(IStringLocalizer<MessagesResources> localizer) { = localizer; _localizer } public string GetGreeting() => _localizer.Get(MessagesResources.Greeting); }
Switch Languages: To test other languages, change the app’s default culture in
Program.cs
.
Automate with RunOnSave
To keep your resources updated without manual re-runs, you can
configure DotLocz to automatically update RESX files when saving a
.loc.csv
file. Here’s how to set this up with
RunOnSave in VSCode:
Install RunOnSave: In VSCode, add the RunOnSave extension.
Set Up Watch Command: Configure the extension to run DotLocz every time you save a
.loc.csv
file:"emeraldwalk.runonsave": { "commands": [ { "match": ".*\\.loc\\.csv$", "cmd": "dot-locz . Locz" } ] }
With this setup, DotLocz will regenerate your RESX files and enums
every time you save a .loc.csv
file, keeping everything in
sync effortlessly.
Benefits in Large Projects
DotLocz is particularly valuable for large, multi-project solutions. Here are some advantages I’ve experienced:
- AI-Friendly Autocompletion: The CSV format supports AI tools, making it easy to expand languages.
- Organized Localization: Separate
.loc.csv
files per module keep resources structured and easy to manage. - Multi-Project Scanning: DotLocz can handle localization across large solutions, generating separate output folders for each project.
- Enjoyable Localization: Adding languages is simple and enjoyable, turning localization from a chore into a smooth process.
See DotLocz in Action
For a full demo, check out the DotLocz Demo on GitHub, featuring a Blazor WebAssembly app with localized default components like Counter and Weather.
Conclusion
DotLocz transformed my own localization process, especially in large, modular projects. If you’re managing multilingual support in .NET, DotLocz might just be the tool to save you time and bring order to your workflow. Explore the tool on GitHub, and let me know how it enhances your localization experience!